如何在 React 中進行樣式設計(CSS in React) | React
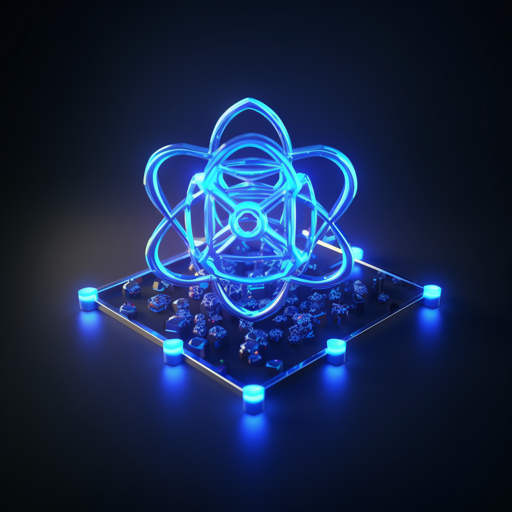
在建立 React 應用程式時,有效的樣式設計是至關重要的一環。React 提供了許多不同的方式來管理樣式,從傳統的 CSS 檔案到各種 CSS-in-JS 解決方案。本篇文章將介紹一些常見的方法,並提供簡單的程式碼範例。
使用 CSS 檔案
最簡單的方法是將 CSS 檔案引入到 React 應用程式中。你可以直接在 index.html
中引入樣式表,或者使用 import
來將樣式表引入到 React 元件中。
App.css
.container {
max-width: 960px;
margin: 0 auto;
padding: 0 20px;
}
.button {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
App.js
import React from 'react';
import './App.css';
function App() {
return (
<div className="container">
<button className="button">Click me</button>
</div>
);
}
export default App;
使用 CSS Modules
CSS Modules 是另一種常見的樣式解決方案,它允許在 React 應用程式中將 CSS 樣式模組化,以避免全域樣式衝突。
Button.module.css
.button {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
App.js
import React from 'react';
import styles from './Button.module.css';
function App() {
return (
<div className={styles.container}>
<button className={styles.button}>Click me</button>
</div>
);
}
export default App;
使用 CSS-in-JS
CSS-in-JS 是一種將 CSS 寫入 JavaScript 檔案的方法,它將樣式和元件直接關聯起來,使得樣式設計更具有彈性和可控性。
安裝styled-components套件
npm install styled-components
可以安裝vscdoe相關套件,增加開發效率
styled-components程式碼範例
import React from 'react';
import styled from 'styled-components';
const Container = styled.div`
max-width: 960px;
margin: 0 auto;
padding: 0 20px;
`;
const Button = styled.button`
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
`;
function App() {
return (
<Container>
<Button>Click me</Button>
</Container>
);
}
export default App;
以上是一些常見的在 React 應用程式中進行樣式設計的方法和範例。你可以根據項目的需求和個人喜好選擇最適合的方式來管理樣式。希望這篇文章對你有所幫助!
Tags