Lists Tuples Sets Dictionary(列表 元組 集合 字典) | Python
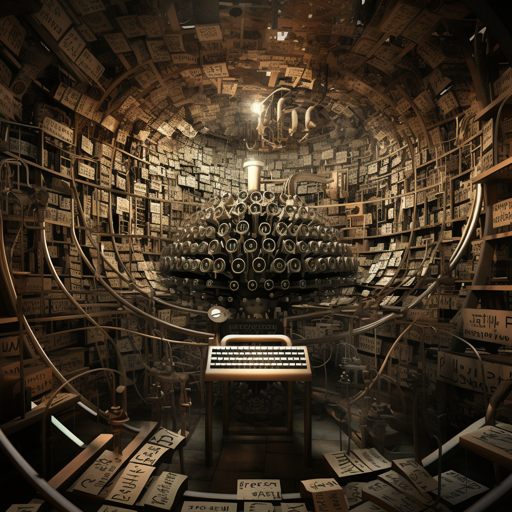
Python 的數據每種結構都針對特定用例而設計。我們這邊利用範例展示 Python 的列表、元組、集合和字典,展示它們如何能夠高效處理數據。
Python 列表(List):
列表是可變的序列,允許添加、刪除或修改元素。
my_list = [1, 2, 3, 'python', True]
my_list.append(4)
print(my_list) # [1, 2, 3, 'python', True, 4]
Python 元組(Tuple):
元組是不可變的序列,確保數據的完整性。一旦定義,元素就不能更改。
my_tuple = (1, 2, 3, 'python', True)
print(my_tuple) # (1, 2, 3, 'python', True)
Python 集合(Set):
集合是無序的唯一元素集合,對於消除重復項目等任務非常有用。
my_set = {1, 2, 3, 'python', True}
my_set.add(4)
print(my_set) # {1, 2, 3, 4, 'python'}
Python 字典(Dictionary):
字典是鍵-值對,提供高效的數據檢索。鍵必須是唯一的。
my_dict = {'name': 'John', 'age': 25, 'city': 'Pythonville'}
my_dict['occupation'] = 'Developer'
print(
my_dict
) # {'name': 'John', 'age': 25, 'city': 'Pythonville', 'occupation': 'Developer'}
範例程式碼:
# 使用列表
my_list = [1, 2, 3]
my_list.append(4) # [1, 2, 3, 4]
my_list.remove(2) # [1, 3, 4]
# 利用元組
my_tuple = (1, 2, 3)
# 嘗試 my_tuple[0] = 5 會導致錯誤
# 發揮集合的作用
my_set = {1, 2, 3, 4, 4}
unique_elements = set(my_list) # {1, 3, 4}
# 探索字典
my_dict = {'name': 'Alice', 'age': 30}
my_dict['occupation'] = 'Designer' # {'name': 'Alice', 'age': 30, 'occupation': 'Designer'}
加強 Python 資料管理:
了解何時使用列表、元組、集合或字典使開發人員能夠開發更高效和有結構的程式碼。
- 列表和元組處理有序序列
- 集合消除重復元素
- 字典擅長基於鍵(唯一值)的資料索引。
Tags