常用的Utility和範例 | TypeScript
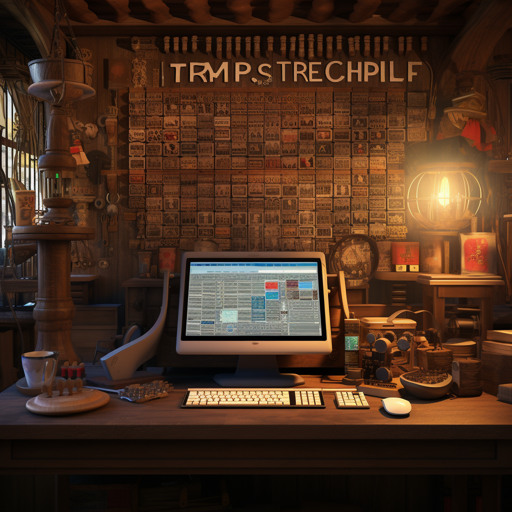
使 TypeScript 的其中一個關鍵特點就是它的 Utility 。我們將介紹20個 TypeScript Utility 和範例,並講解使用的優勢。
理解 TypeScript Utility
TypeScript 泛型(Generics) 和 實用(Utility) 型別,在 TypeScript 的核心是泛型(Generics)的概念,提供了代碼的靈活性和可重用性。實用型別則補充了泛型,為常見的類型操作提供了預定好的工具。
1. Partial - 使屬性可選
Partial 實用型別允許我們使型別 T 中的所有屬性都變為可選。
這讓我們在處理數據結構時保有原本物件的靈活性。
interface User {
name: string;
age: number;
}
const partialUser: Partial<User> = { name: 'John' };
2. Required - 確保必填屬性
相反,Required 確保型別 T 中的所有屬性都是必須的。
interface User {
name?: string;
age?: number;
}
const requiredUser: Required<User> = { name: 'John', age: 30 };
3. Readonly - 不可變性保證
Readonly 將型別 T 中的所有屬性轉換為唯讀,使其成為不可變。
interface Configuration {
apiKey: string;
endpoint: string;
}
const readOnlyConfig: Readonly<Configuration> = { apiKey: '123', endpoint: 'https://example.com' };
4. Record<K, T> - 物件鍵值對
Record 實用型別創建一個帶有特定鍵值對的物件,促使以結構化的方式存儲數據。
type Fruit = 'apple' | 'banana' | 'orange';
const fruitCount: Record<Fruit, number> = { apple: 5, banana: 3, orange: 8 };
5. Pick<T, K> - 選擇特定屬性
使用 Pick,從一個型別中選擇特定的屬性。
interface User {
name: string;
age: number;
address: string;
}
const pickedUser: Pick<User, 'name' | 'age'> = { name: 'John', age: 30 };
6. Omit<T, K> - 排除特定屬性
Omit 實用型別則相反,從一個型別中排除特定的屬性。
interface User {
name: string;
age: number;
address: string;
}
const omittedUser: Omit<User, 'address'> = { name: 'John', age: 30 };
進階 TypeScript Utility:
1.Exclude<T, U> - 從聯合類型中排除類型
Exclude 可以從一個聯合類型中過濾掉某些類型,提供了一種簡潔的方式來縮小選擇範圍。
type Numbers = 1 | 2 | 3 | 4 | 5;
type NonEvenNumbers = Exclude<Numbers, 2 | 4>;
2. Extract<T, U> - 从联合类型中提取类型
相反的,Extract可以從聯合類型中提取特定的類型,提高代碼的靈活性。
type Numbers = 1 | 2 | 3 | 4 | 5;
type EvenNumbers = Extract<Numbers, 2 | 4>;
3. NonNullable - 消除 null 和 undefined
NonNullable 排除了 null 和 undefined。
type NullableString = string | null | undefined;
type NonNullableString = NonNullable<NullableString>;
4. ReturnType - 捕獲函數返回類型
使用 ReturnType,返回函數的類型。
function greet(): string {
return 'Hello, TypeScript!';
}
type Greeting = ReturnType<typeof greet>; // string
結論:
通過採用TypeScript Utility 開發人員可以提升程式碼類型安全性,並簡化複雜的型別操作。大大減低開發時間和讓你的代碼看起來更簡潔優雅。
Tags