常見 JavaScript 字串操作方法總覽 | Javascript
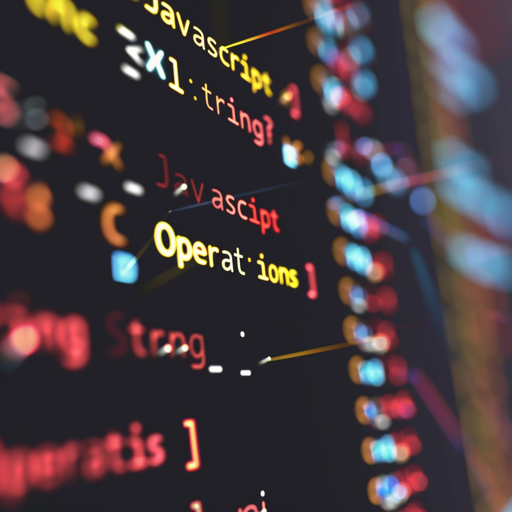
JavaScript 是一門廣泛用於網頁開發的程式語言,它提供了豐富的字串操作方法,讓開發者可以輕鬆地處理和轉換文字數據。
在這篇文章中,我們將深入探討 JavaScript 中一些常用的字串操作方法,讓我們逐一來看看它們的功能和使用方法。
包括:
- indexOf()
- lastIndexOf()
- toLowerCase()
- toUpperCase()
- trim()
- replace()
- replaceAll()
- includes()
- startsWith()
- endsWith()
- split()
- padStart()
- padEnd()
- slice()
- repeat()
1. indexOf()
indexOf()
方法返回指定字串在原字串中首次出現的位置索引,如果找不到,則返回 -1。
const str = 'Hello, world!';
console.log(str.indexOf('o')); // Output: 4
2. lastIndexOf()
lastIndexOf()
方法返回指定字串在原字串中最後一次出現的位置索引,如果找不到,則返回 -1。
const str = 'Hello, world!';
console.log(str.lastIndexOf('o')); // Output: 8
3. toLowerCase()
toLowerCase()
方法將字串轉換為小寫。
const str = 'Hello, World!';
console.log(str.toLowerCase()); // Output: hello, world!
4. toUpperCase()
toUpperCase()
方法將字串轉換為大寫。
const str = 'Hello, World!';
console.log(str.toUpperCase()); // Output: HELLO, WORLD!
5. trim()
trim()
方法刪除字串兩端的空格。
const str = ' Hello, World! ';
console.log(str.trim()); // Output: Hello, World!
6. replace()
replace()
方法替換字串中的匹配項。
const str = 'Hello, World!';
console.log(str.replace('World', 'Universe')); // Output: Hello, Universe!
7. replaceAll()
replaceAll()
方法替換字串中的所有匹配項。
const str = 'Hello, World!';
console.log(str.replaceAll('o', '0')); // Output: Hell0, W0rld!
8. includes()
includes()
方法檢查字串是否包含指定的子字串,並返回 true 或 false。
const str = 'Hello, World!';
console.log(str.includes('World')); // Output: true
9. startsWith()
startsWith()
方法檢查字串是否以指定的子字串開頭,並返回 true 或 false。
const str = 'Hello, World!';
console.log(str.startsWith('Hello')); // Output: true
10. endsWith()
endsWith()
方法檢查字串是否以指定的子字串結尾,並返回 true 或 false。
const str = 'Hello, World!';
console.log(str.endsWith('World!')); // Output: true
11. split()
split()
方法將字串分割成字串陣列。
const str = 'apple,banana,orange';
console.log(str.split(',')); // Output: ['apple', 'banana', 'orange']
12. padStart()
padStart()
方法用指定字元填充字串,以使結果字串達到指定的長度,從開頭填充。
const str = '7';
console.log(str.padStart(3, '0')); // Output: 007
13. padEnd()
padEnd()
方法用指定字元填充字串,以使結果字串達到指定的長度,從結尾填充。
const str = '7';
console.log(str.padEnd(3, '0')); // Output: 700
14. slice()
slice()
方法返回字串的一部分,提取從開始索引到結束索引(不包括結束索引)之間的字串。
const str = 'Hello, World!';
console.log(str.slice(7, 12)); // Output: World
15. repeat()
repeat()
方法返回包含指定數量重複字串的新字串。
const str = 'Hello';
console.log(str.repeat(3)); // Output: HelloHelloHello
這些是 JavaScript 中一些常用的字串操作方法。通過熟練使用這些方法,你可以更輕鬆地處理和操作字串數據,使你的 JavaScript 開發更加高效和便捷。希望這篇文章能夠對你有所幫助!
Tags