this | Javascript
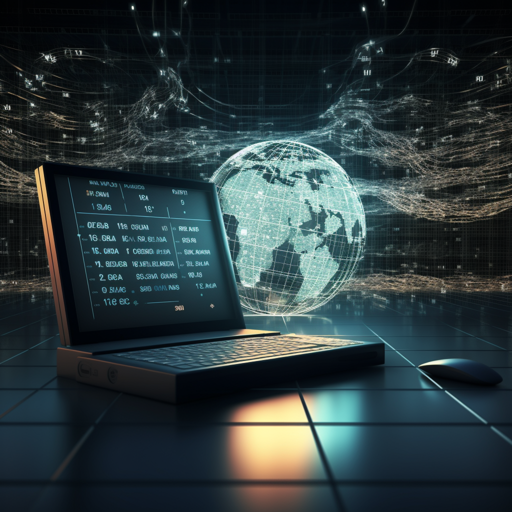
JavaScript 中的 this 既強大又神秘,常常讓開發人員感到困惑。我們將用範例深入探討 this 在不同情境中的運作方式。
理解不同情境中的 this :
- this 的行為取決於它的使用上下文。
- 在全局範圍或函數內部, this 指向全局對象或在嚴格模式下為undefined。
- 在物件方法內部, this 指向對象本身。
- this 是動態的,this 會指向是誰調用它的
- this 只能指向物件,不能指向函數,如果指向函數,this會變成 undefined
- 箭頭函數沒有自己的 this , 它繼承自父級作用域的 this
// 全局上下文
console.log(this); // Window對象
function exampleFunction() {
console.log(this); // Window對象或在嚴格模式下為undefined
}
// 對象上下文
const exampleObject = {
property: '我是對象屬性',
logProperty: function () {
console.log(this.property); // 訪問對象屬性
},
};
exampleObject.logProperty();
箭頭函數和 this :
- 箭頭函數對於 this 有一個獨特的行為。
- 與常規函數不同,箭頭函數沒有自己的 this 上下文。
- 相反,它們繼承自父級作用域的 this 。
- 我們在物件中寫方法的時候,為了避免使用 this 時發生錯誤,我們應該都使用常規函數,而不使用箭頭函數。
function regularFunction() {
console.log(this); // Window對象(Window對象或在嚴格模式下為undefined)
}
const arrowFunction = () => {
console.log(this); // 繼承自父級作用域的"this"
};
事件處理程序和 this :
在事件處理程序中,例如與HTML元素一起使用的事件, this 通常指向觸發事件的元素。
const buttonElement = document.getElementById('myButton');
buttonElement.addEventListener('click', function () {
console.log(this); // 按鈕元素
});
Tags