Truthy and Falsy(真值、假值) | Javascript
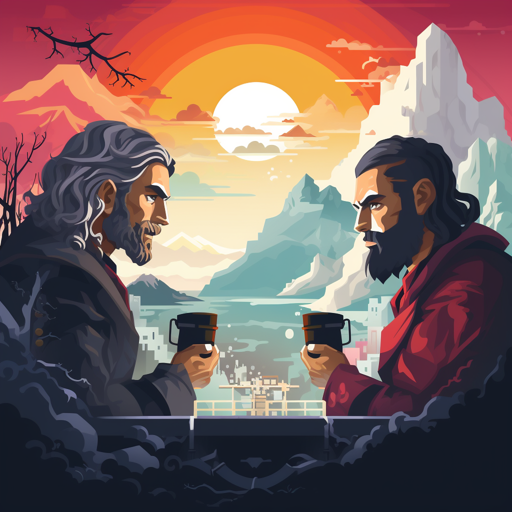
了解真值(例如,字串、數字)和假值(例如,null、undefined)對於編寫高效且清晰的程式碼至關重要。透過實際範例列出常見的真值(Truthy)和假值(Falsy)場景,很好的幫助你寫出高效的程式碼。
了解 JavaScript 的 真值(Truthy) 和 假值(Falsy) 對於編寫靈活的程式碼非常重要。在 JavaScript 中,每個值都有固有的真值(Truthy)和假值(Falsy),影響條件語句。假值包括 0 、 "" 、 undefined 、 null 、NaN 和 false,而所有其他值都為真值。
判斷 true or false 在控制流(如 if-else 語句)中可以讓我們靈活得開發 JavaScript。例如,在檢查變數是否具有值時,JavaScript 隱含會評估其是否為真值。了解值的真值(Truthy)和假值(Falsy)能使開發人員能夠寫出高效的程式碼,並且提高程式碼的可讀性和邏輯性。
這篇文章透過實際範例列出常見的真值(Truthy)和假值(Falsy)場景。無論你是初學者還是經驗豐富的開發人員,掌握這些概念都能增強你編寫乾淨且高效的 JavaScript 程式碼。
See the Pen Truthy and Falsy(真值、假值)-Javascript by lenrich (@lenrich) on CodePen.
範例
'use strict';
console.log(Boolean(0)); // false
console.log(Boolean(undefined)); // false
console.log(Boolean('Jeter')); // true
console.log(Boolean({})); // true
console.log(Boolean('')); // false
const temperature = 25;
if (temperature) {
console.log('The weather is just right!');
} else {
console.log('Bring a jacket, it might be cold.');
}
// The weather is just right!
let username; // undefined
if (username) {
console.log(`Welcome, ${username}!`);
} else {
console.log('Please log in to continue.');
}
// Please log in to continue.
const fruits = [];
if (fruits) {
console.log('You have some delicious fruits!');
} else {
console.log('Your fruit basket is empty.');
}
// You have some delicious fruits!
let isStudent = true;
if (isStudent) {
console.log('Keep up the good work in your studies!');
} else {
console.log('Consider enrolling in a course.');
}
// Keep up the good work in your studies!
Tags