Type Conversion(型別轉換) | Javascript
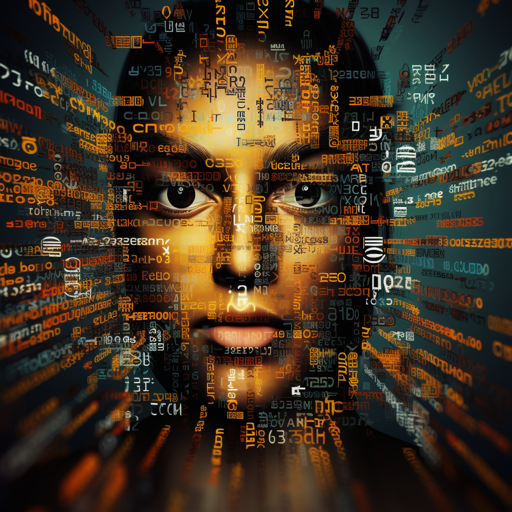
理解 JavaScript 如何進行型別轉換和強制轉換非常重要。這就是為什麼一個字串
5
可以變成數字 5 的原因。在這篇文章中,我們將示範 JavaScript 如何進行這些轉換,讓你簡單的理解運作的原理。
JavaScript 類型轉換和強制轉換是靈活處理不同資料類型的方式。類型轉換是更改資料類型,而強制轉換是語言在操作期間隱式轉換類型的方式。自動將數字類型轉換成字串類型。這篇部落格文章將示範 JavaScript 如何在不同資料型別之間靈活切換,解釋顯式和隱式轉換。透過更深入理解類型轉換和強制轉換來深入理解 JavaScript 的類型!
See the Pen Type Conversion(型別轉換)-Javascript by lenrich (@lenrich) on CodePen.
範例
'use strict';
// type conversion
const userInput = '5.89';
console.log(Number(userInput), userInput); // 5.89 '5.89' (Convert string to number)
console.log(Number(userInput) * 2); // 11.78 (Perform numerical operations)
console.log(Number('Alice')); // NaN
console.log(typeof NaN); // number
console.log(typeof new String('hello')); // object
console.log(typeof String(true)); // string
console.log(typeof new Number('42')); // object
console.log(typeof Number(true)); // number
console.log(String(false), false); // false false (Convert boolean to string)
// type coercion
console.log('The answer is ' + 42); // 'The answer is 42' (Combine string and number)
console.log('100' - '10' + 5); // 95 (Perform arithmetic operations)
console.log('6' / '3'); // 2 (Divide strings)
let result = '2' * 3; // 6
result = result + ' apples'; // '6 apples'
console.log(result);
let x = '5' - 2; // 3
x = x + ' bananas'; // '3 bananas'
console.log(x);
let y = '5' + 2; // '52'
y = y - 1;
console.log(y); // 51
Tags