Template Literals(字串模板) | Javascript
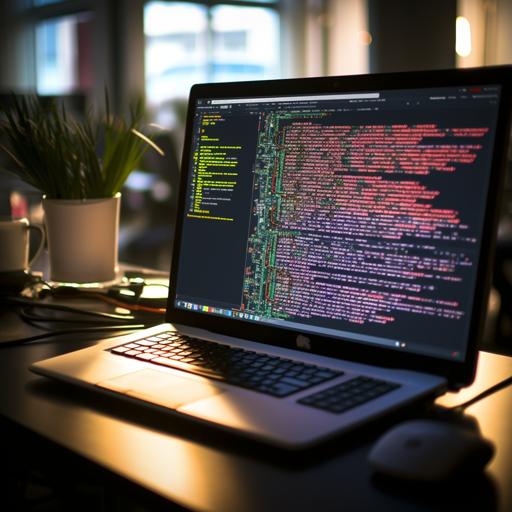
Template Literals(字串模板) ,在 Javascript 中我們會很常用使用到,在字串(string)中帶入變數(variable)的技巧一定要熟練的掌握。
字串模板是為了讓傳統的字串拼接不用在每個變數中使用+,這樣程式碼會變得很混亂不易閱讀 使用字串模板可以快速進行字串拼接
See the Pen Template Literals(字串模板)-Javascript by lenrich (@lenrich) on CodePen.
範例
'use strict';
const dogName = 'Buddy';
const dogBreed = 'Golden Retriever';
const dogBirthYear = 2015;
const currentYear = 2023;
// Using string concatenation
const dogInfo =
'Meet ' + dogName + ', a ' + (currentYear - dogBirthYear) + '-year-old ' + dogBreed + '!';
console.log(dogInfo); // Meet Buddy, a 8-year-old Golden Retriever!
// Using template literals
const dogInfoNew = `Meet ${dogName}, a ${currentYear - dogBirthYear}-year-old ${dogBreed}!`;
console.log(dogInfoNew); // Meet Buddy, a 8-year-old Golden Retriever!
// Simple template literals
console.log(`Just a simple string...`); // Just a simple string...
// Multi-line strings using string concatenation
console.log('String with\n' + 'multiple\n' + 'lines');
// String with
// multiple
// lines
// Multi-line strings using template literals
console.log(`
String with
multiple
lines
`);
//
// String with
// multiple
// lines
Tags